Posted: Dezember 21st, 2012 | Filed under: Java, Programmieren | Tags: Beispiel, Countdown, Example, Java, Swing, Timer, Tutorial | 12 Comments »
Häufig benötigt man für Applikationen einen Zeitnehmer, oder es soll ein bestimmtes Event nach einer gewissen Zeit ausgeführt werden. Mit Hilfe der Klasse Timer aus der Swing-Bibliothek kann ein sogenannter Countdown leicht erstellt werden. Das folgende Beispiel veranschaulicht die Realisierung eines Countdowns.
Screenshot
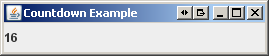
Quellcode
import java.awt.BorderLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.Timer; import javax.swing.WindowConstants; public class Countdown extends JFrame { // Countdown mit 42 Sekunden public static int counterValue = 42; public static Timer timer; public static JLabel label; public Countdown() { initGUI(); } // GUI-Erzeugen private void initGUI(){ BorderLayout thisLayout = new BorderLayout(); this.setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE); this.getContentPane().setLayout(thisLayout); label = new JLabel(); label.setText(String.valueOf(counterValue)); this.getContentPane().add(label, BorderLayout.CENTER); this.setTitle("Countdown Example"); this.pack(); this.setVisible(true); } public static void main(String[] args) { // GUI erzeugen Countdown countdown = new Countdown(); // Timer erzeugen, jede 1000 Millisekunden (= 1 Sekunde) // Methode actionPerformed aufrufen. Countdown.timer = new Timer(1000, new ActionListener() { public void actionPerformed(ActionEvent e) { // 1 Sekunde abziehen Countdown.counterValue--; // Zahl in Label darstellen Countdown.label.setText(String.valueOf(counterValue)); // Falls Zähler = 0, Countdown abgelaufen! if(Countdown.counterValue == 0){ System.out.println("Counterdown ausgelaufen!"); // Timer stoppen Countdown.timer.stop(); } } }); // Timer starten timer.start(); } } |
In der Klasse Timer kann im Konstruktor ein Zeitwert und ein Listener übergeben werden. Die actionPerformed-Methode dieses Listeners wird, nachdem der Timer gestartet wurde, alle 1000 ms (=1 s), aufgerufen. Die Methode actionPerfomed ist also unser Taktgeber beim Abziehen einer Sekunde von der aktuellen Countdown-Zahl. Erreicht unsere Zahl den Wert 0, ist der Countdown schließlich abgelaufen.
Drei ist die sicherste und genaueste Ansatz, meiner Meinung nach. Sie können machen Ihre Anwendung reagieren zu fühlen, indem sichtlich darauf hinweist, dass von Daten auf das erste Resize-Ereignis laden, und passen Sie den Countdown timer um das Gefühl Ihrer Anwendung richtig.
I dont get it
bin gerade am java lernen und hab mal rumgespielt mit deinem code. Gefällt mir – danke 🙂
Vielen Dank fürs positive Feedback!
I noticed your page’s ranking in google’s search results is very low.
You are loosing a lot of traffic. You need hi authority backlinks to rank in top10.
I know – buying them is too expensive. It is better to own them.
I know how to do that, simply google it:
Polswor’s Backlinks Source
danle für diesen CODE
WEITER SO!
#pennisarmy
Hello admin i see you don’t earn on your page. You can earn extra money easily, search
on youtube for: how to earn selling articles
I have noticed you don’t monetize your page, don’t waste your traffic, you can earn extra cash every month because you’ve
got high quality content. If you want to know how to make extra
bucks, search for: Mertiso’s tips best adsense alternative
I have checked your website and i have found some duplicate content, that’s why you don’t rank high in google,
but there is a tool that can help you to create 100% unique
content, search for: Boorfe’s tips unlimited content
I have checked your page and i’ve found some duplicate content,
that’s why you don’t rank high in google, but there is a tool that can help you to create 100% unique
articles, search for: SSundee advices unlimited content for your
blog
I have noticed you don’t monetize your page, don’t waste your traffic, you can earn extra bucks
every month. You can use the best adsense alternative for any type of website
(they approve all websites), for more info simply search in gooogle: boorfe’s tips monetize your website
May I just say what a comfort to discover someone who really
understands what they’re talking about on the web. You certainly
realize how to bring a problem to light and make it important.
More people need to read this and understand this side of the story.
I can’t believe you aren’t more popular because you certainly possess
the gift.